This tutorial will show you how to send WhatsApp messages from ESP8266 NodeMCU board. This may be useful to receive sensor reading alerts from the ESP8266, alert messages when a sensor reading is over or below a certain threshold, and many more uses. The ESP8266 will be programmed using the Arduino IDE, and CallMeBot's free API will be used to transmit the messages.
Introducing WhatsApp
“WhatsApp Messenger, or simply WhatsApp, is an American cross-platform, freeware, centralized instant messaging and voice-over-IP service that is available internationally and is owned by Meta Platforms.” You may avoid paying SMS rates by using your phone's internet connection to send messages.
Available on iOS and Android, WhatsApp is free. If you don't already have WhatsApp on your smartphone, get it now.
CallMeBot WhatsApp API
We'll use an unrestricted API service called CallMeBot to send WhatsApp messages from ESP8266 NodeMCU board. Visit the following website to learn more about CallMeBot:
It works as a gateway that allows you to send yourself a message. Sending alert messages from the ESP8266 using this might be useful.
You can get all the information on how to send messages using the API, which can be found here.
Read More: Install ESP8266 Board in Arduino IDE in less than 1 minute
Getting the CallMeBot API Key
You need to get the CallmeBot WhatsApp API key before starting to use the API. To see the next set of instructions on the official website, click this link.
- Add the phone number +34 644 44 21 48 to your phone contacts. (You may give it any name).
- Send the following message to the newly established contact: “I allow callmebot to send me messages” (of course using WhatsApp).
- Await the bot's message, “API Activated for Your Phone Number. Your API KEY is XXXXXXX“.
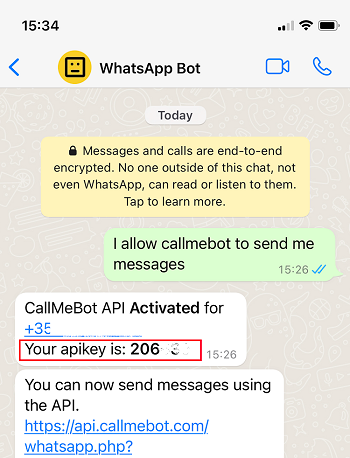
Note: Please try again after 24 hours if you don't receive the API key within 2 minutes. The API key needed to send messages using the API will be included in the WhatsApp message sent by the bot.
CallMeBot API
You need to send a POST request with your information to the following URL in order to send a message using the CallMeBot API:
https://api.callmebot.com/whatsapp.php?phone=[phone_number]&text=[message]&apikey=[your_apikey]
- [phone_number]: phone number associated with your WhatsApp account in international format.
- [message]: The message to be sent should be URL-encoded.
- [your_apikey]: the API key you received during the activation process in the previous section.
You may click on the following link for the official documentation: https://www.callmebot.com/blog/free-api-whatsapp-messages/
Installing the URLEncode Library
The message that has to be sent must be URL-encoded, as we've seen before. Characters are changed by URL encoding so they may be transmitted over the Internet. Only using the ASCII character set can URLs be sent over the Internet.
In our messages, this will allow us to include characters like ç, ª, º, à, ü. URL encoding is something you can learn more about here.
You may either encode the message manually or use a library, which is much simpler. The UrlEncode library, which can be installed on your Arduino IDE, will be used.
Search for the URLEncode library by Masayuki Sugahara under Sketch > Include Library > Manage Libraries, as shown below.
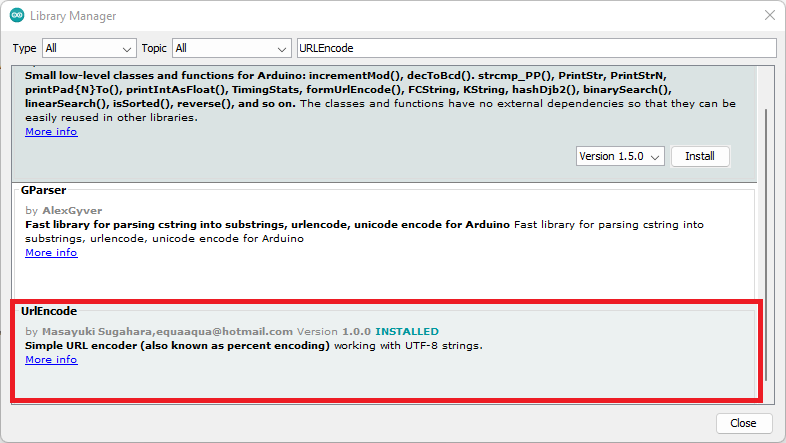
Sending Messages to WhatsApp: ESP8266 Code
When the ESP8266 first boots, the following example code sends a message to your WhatsApp account. This is a simple example that will show you how to send messages. Understanding how it works is the first step to implementing it in your own projects.
/*
LEDEdit PRO
Complete project details at https://lededitpro.com/send-whatsapp-messages-from-esp8266-nodemcu/
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files.
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
*/
#include <ESP8266WiFi.h>
#include <ESP8266HTTPClient.h>
#include <WiFiClient.h>
#include <UrlEncode.h>
const char* ssid = "REPLACE_WITH_YOUR_SSID";
const char* password = "REPLACE_WITH_YOUR_PASSWORD";
// +international_country_code + phone number
// Portugal +351, example: +351912345678
String phoneNumber = "REPLACE_WITH_YOUR_PHONE_NUMBER";
String apiKey = "REPLACE_WITH_API_KEY";
void sendMessage(String message){
// Data to send with HTTP POST
String url = "http://api.callmebot.com/whatsapp.php?phone=" + phoneNumber + "&apikey=" + apiKey + "&text=" + urlEncode(message);
WiFiClient client;
HTTPClient http;
http.begin(client, url);
// Specify content-type header
http.addHeader("Content-Type", "application/x-www-form-urlencoded");
// Send HTTP POST request
int httpResponseCode = http.POST(url);
if (httpResponseCode == 200){
Serial.print("Message sent successfully");
}
else{
Serial.println("Error sending the message");
Serial.print("HTTP response code: ");
Serial.println(httpResponseCode);
}
// Free resources
http.end();
}
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
Serial.println("Connecting");
while(WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.print("Connected to WiFi network with IP Address: ");
Serial.println(WiFi.localIP());
// Send Message to WhatsAPP
sendMessage("Hello from ESP8266!");
}
void loop() {
}
Read More: Build an ESP8266 Web Server: The Complete Guide
How the Code Works
Using the CallMeBot API, sending a message to WhatsApp is simple. You just need to send an HTTP POST request.
First, include the necessary libraries:
#include <ESP8266WiFi.h>
#include <ESP8266HTTPClient.h>
#include <WiFiClient.h>
#include <UrlEncode.h>
Insert your network credentials into the following variables:
const char* ssid = "REPLACE_WITH_YOUR_SSID";
const char* password = "REPLACE_WITH_YOUR_PASSWORD";
Add your API key and phone number. The phone number has to be in international format, (including the + sign).
String phoneNumber = "REPLACE_WITH_YOUR_PHONE_NUMBER";
String apiKey = "REPLACE_WITH_YOUR_API_KEY";
sendMessage()
To send messages to WhatsApp, we create a function called sendMessage()
that you may call later. This function takes the message you wish to send as an argument.
void sendMessage(String message){
We prepare the URL for the request inside the function using your information, phone number, API key, and message.
The message needs to be URL-encoded, as we've seen earlier. To do this, we've included the UrlEncode
library. It has a function called urlEncode()
that encodes any message sent as an argument (urlEncode(message)
).
String url = "http://api.callmebot.com/whatsapp.php?phone=" + phoneNumber + "&apikey=" + apiKey + "&text=" + urlEncode(message);
Create and start an HTTPClient
on that URL:
HTTPClient http;
http.begin(url);
Specify the content type:
// Specify content-type header
http.addHeader("Content-Type", "application/x-www-form-urlencoded");
Send the HTTP post request when you're done. The following line both sends the request and saves the response code:
int httpResponseCode = http.POST(url);
When the response code is 200, it means that the post request was successful. Something went wrong elsewhere.
// Send HTTP POST request
int httpResponseCode = http.POST(url);
if (httpResponseCode == 200){
Serial.print("Message sent successfully");
}
else{
Serial.println("Error sending the message");
Serial.print("HTTP response code: ");
Serial.println(httpResponseCode);
}
Finally, free up the resources:
// Free resources
http.end();
setup()
In setup(), initialize the serial monitor for debugging purposes.
Serial.begin(115200);
Connect to your local network and print the board's IP address.
WiFi.begin(ssid, password);
Serial.println("Connecting");
while(WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.print("Connected to WiFi network with IP Address: ");
Serial.println(WiFi.localIP());
Then, by simply calling the sendMessage() function, we can send a message to WhatsApp. In this case, we're sending the Hello message from the ESP8266!
// Send Message to WhatsAPP
sendMessage("Hello from ESP8266!");
Read More: ESP8266 NodeMCU With BH1750 Ambient Light Sensor Using Arduino
Demonstration
You may upload the code to your board after inserting your network credentials, phone number, and API key.
After uploading, open the serial monitor at 115200 baud rate and press the RST button on the board. It should connect to your network and send the message to WhatsApp successfully.
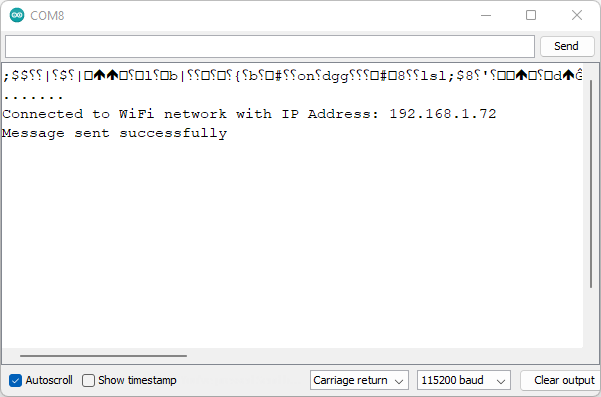
Go to your WhatsApp account. You should receive the ESP8266 message in a few seconds.
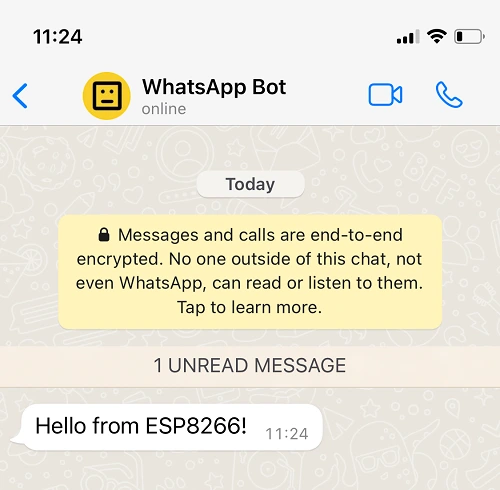
Conclusion
In this tutorial, you learned how to use the CallMeBot API with the ESP8266 to send messages to your WhatsApp account. This may be used to send sensor readings regularly to your mailbox, to send a notification when motion is detected, to send an alert message when a sensor reading is above or below a specified threshold, and for a variety of other purposes.
We hope you find this tutorial useful. Thanks for reading.
To code works fine but I have getting error in sending messages to whataspp keeps saying error sending messages.
I have entered the right whatbot credentials..please help with an example how to modify the string url with my WhatsApp number..