Using the Arduino IDE, you'll learn how to set up an ESP8266 NodeMCU access point for a web server. This enables you to connect to your ESP8266 through Wi-Fi without the need for a wireless router.
To set the ESP8266 as an access point, use WiFi.softAP(ssid, password);
ESP8266 Station and Access Point
We connect the ESP8266 to a wireless router in most of our ESP8266 NodeMCU web server projects. In this configuration, we may connect to the ESP8266 over the local network.
In this case, the ESP8266 is set up as a station, while the router serves as an access point. So, to control the ESP8266, you need to be connected to your router's local network.
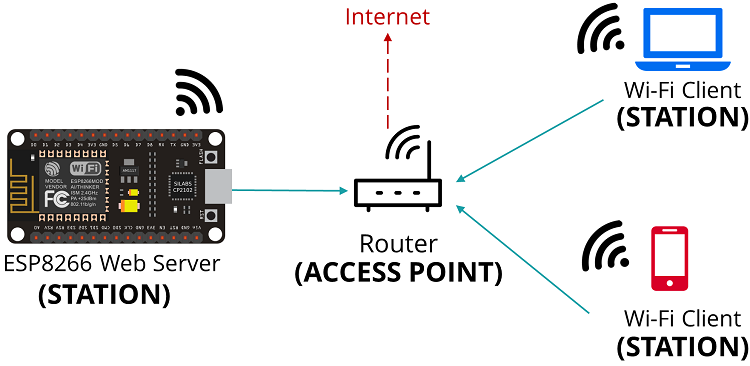
In some cases (when you don't have a router nearby), this may not be the ideal option. However, if you set the ESP8266 up as an access point (hotspot), you may connect to it using any device that supports Wi-Fi without the need to connect to your router.
In simple terms, when you set the ESP8266 as an access point, you create its own Wi-Fi network, and nearby Wi-Fi devices (stations) may connect to it.
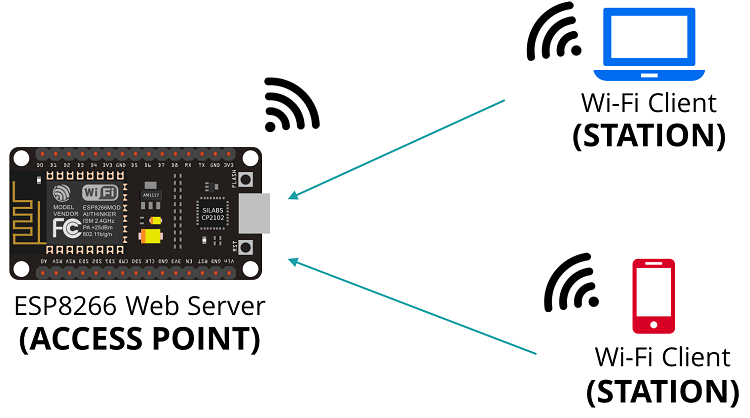
In this article, we'll show you how to set up the ESP8266 as an access point in your web server projects. You don't need to be connected to a router to control your ESP8266 this way.
The ESP8266 is called a soft-AP (soft Access Point) since it does not connect to a wired network (like your router).
This means that trying to load libraries or use firmware via the internet will not work. It also doesn't work when you try to send HTTP requests to internet services, such as publishing sensor readings to the cloud.
Installing the DHT Library for ESP8266
We'll use a previous web server project that displays sensor readings from a DHT sensor for this example.
To read from the DHT sensor, we'll use the Adafruit DHT library with the ESP8266 board installed in the Arduino IDE. You will also need to install the Adafruit Unified Sensor library to use this library.
To install the two libraries, follow the next steps:
1. Open your Arduino IDE and go to Sketch > Include Library > Manage Libraries. The library manager should open.
2. Search for “DHT” in the search box and install the DHT library from Adafruit.
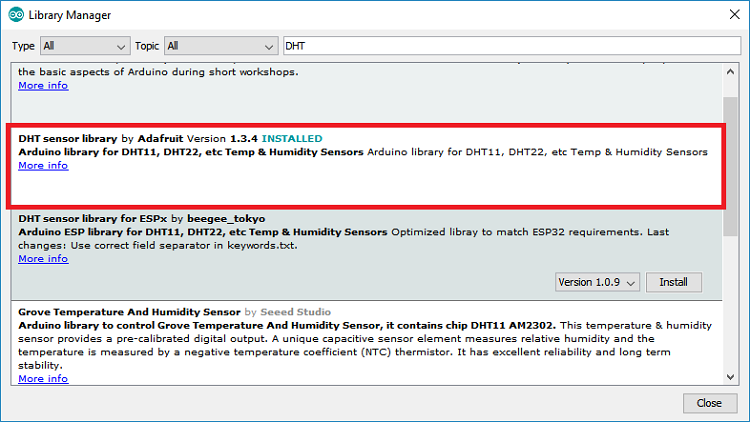
After installing the Adafruit DHT library, search for “Adafruit Unified Sensor” in the search box. Scroll all the way down to find and install the library.
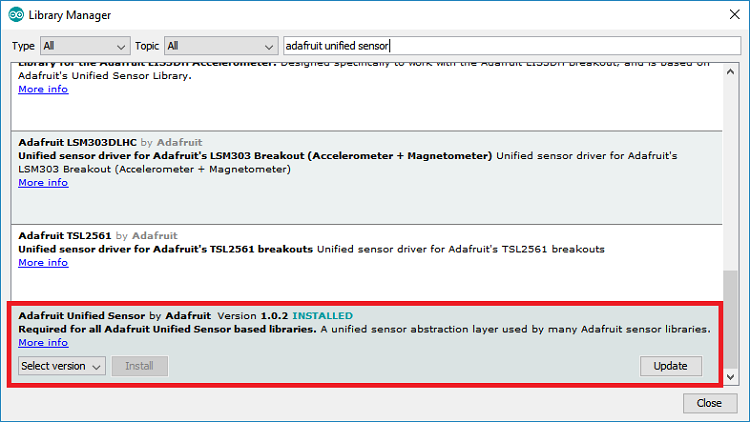
After installing the libraries, restart your Arduino IDE.
ESP8266 NodeMCU Access Point (AP)
In this example, we'll modify the ESP8266 Web Server from a previous tutorial to add access point functionality. Here's an example of a project we'll use: ESP8266 DHT11/DHT22 Temperature and Humidity Web Server with Arduino IDE
What we're going to show you here may be used with any ESP8266 web server example.
To set the ESP8266 as an access point, upload the sketch provided below.
/*********
LEDEdit PRO
Complete project details at https://lededitpro.com/esp8266-nodemcu-access-point-for-a-web-server/
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files.
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
*********/
// Import required libraries
#include <Arduino.h>
#include <ESP8266WiFi.h>
#include <Hash.h>
#include <ESPAsyncTCP.h>
#include <ESPAsyncWebServer.h>
#include <Adafruit_Sensor.h>
#include <DHT.h>
const char* ssid = "ESP8266-Access-Point";
const char* password = "123456789";
#define DHTPIN 5 // Digital pin connected to the DHT sensor
// Uncomment the type of sensor in use:
//#define DHTTYPE DHT11 // DHT 11
#define DHTTYPE DHT22 // DHT 22 (AM2302)
//#define DHTTYPE DHT21 // DHT 21 (AM2301)
DHT dht(DHTPIN, DHTTYPE);
// current temperature & humidity, updated in loop()
float t = 0.0;
float h = 0.0;
// Create AsyncWebServer object on port 80
AsyncWebServer server(80);
// Generally, you should use "unsigned long" for variables that hold time
// The value will quickly become too large for an int to store
unsigned long previousMillis = 0; // will store last time DHT was updated
// Updates DHT readings every 10 seconds
const long interval = 10000;
const char index_html[] PROGMEM = R"rawliteral(
<!DOCTYPE HTML><html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>
html {
font-family: Arial;
display: inline-block;
margin: 0px auto;
text-align: center;
}
h2 { font-size: 3.0rem; }
p { font-size: 3.0rem; }
.units { font-size: 1.2rem; }
.dht-labels{
font-size: 1.5rem;
vertical-align:middle;
padding-bottom: 15px;
}
</style>
</head>
<body>
<h2>ESP8266 DHT Server</h2>
<p>
<span class="dht-labels">Temperature</span>
<span id="temperature">%TEMPERATURE%</span>
<sup class="units">°C</sup>
</p>
<p>
<span class="dht-labels">Humidity</span>
<span id="humidity">%HUMIDITY%</span>
<sup class="units">%</sup>
</p>
</body>
<script>
setInterval(function ( ) {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("temperature").innerHTML = this.responseText;
}
};
xhttp.open("GET", "/temperature", true);
xhttp.send();
}, 10000 ) ;
setInterval(function ( ) {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("humidity").innerHTML = this.responseText;
}
};
xhttp.open("GET", "/humidity", true);
xhttp.send();
}, 10000 ) ;
</script>
</html>)rawliteral";
// Replaces placeholder with DHT values
String processor(const String& var){
//Serial.println(var);
if(var == "TEMPERATURE"){
return String(t);
}
else if(var == "HUMIDITY"){
return String(h);
}
return String();
}
void setup(){
// Serial port for debugging purposes
Serial.begin(115200);
dht.begin();
Serial.print("Setting AP (Access Point)…");
// Remove the password parameter, if you want the AP (Access Point) to be open
WiFi.softAP(ssid, password);
IPAddress IP = WiFi.softAPIP();
Serial.print("AP IP address: ");
Serial.println(IP);
// Print ESP8266 Local IP Address
Serial.println(WiFi.localIP());
// Route for root / web page
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/html", index_html, processor);
});
server.on("/temperature", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/plain", String(t).c_str());
});
server.on("/humidity", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/plain", String(h).c_str());
});
// Start server
server.begin();
}
void loop(){
unsigned long currentMillis = millis();
if (currentMillis - previousMillis >= interval) {
// save the last time you updated the DHT values
previousMillis = currentMillis;
// Read temperature as Celsius (the default)
float newT = dht.readTemperature();
// Read temperature as Fahrenheit (isFahrenheit = true)
//float newT = dht.readTemperature(true);
// if temperature read failed, don't change t value
if (isnan(newT)) {
Serial.println("Failed to read from DHT sensor!");
}
else {
t = newT;
Serial.println(t);
}
// Read Humidity
float newH = dht.readHumidity();
// if humidity read failed, don't change h value
if (isnan(newH)) {
Serial.println("Failed to read from DHT sensor!");
}
else {
h = newH;
Serial.println(h);
}
}
}
Customize the SSID and Password
To access the ESP8266, you will need to define an SSID name and a password. In this example, we're setting the ESP8266 SSID to ESP8266-Access-Point
, but you may modify the name to whatever you wish. However, you may modify the password, which is 123456789
.
const char* ssid = "ESP8266-Access-Point";
const char* password = "123456789";
Setting the ESP8266 as an Access Point (AP)
There is a section in setup()
that describes how to configure the ESP8266 as an access point using the softAP()
method:
WiFi.softAP(ssid, password);
There are other optional parameters that may be sent to the softAP()
function. All of the parameters are listed below:
.softAP(const char* ssid, const char* password, int channel, int ssid_hidden, int max_connection)
ssid
(defined earlier): maximum of 31 characterspassword
(defined earlier): minimum of 8 characters. If not specified, the access point will be open (maximum 63 characters)channel
: Wi-Fi channel number (1-13). Default is 1ssid_hidden
: if set to true will hide SSIDmax_connection
: max simultaneous connected stations, from 0 to 8
Then, using the softAPIP()
method, get the access point IP address and print it in the Serial Monitor.
IPAddress IP = WiFi.softAPIP();
Serial.print("AP IP address: ");
Serial.println(IP);
Note: by default, the access point IP address is 192.168.4.1
To set up the ESP8266 as a soft access point, you need to include these code snippets in your web server sketches.
Parts Required
You will need the following items to proceed with this project:
- ESP8266 development board
- DHT22 or DHT11 Temperature and Humidity Sensor
- 4.7k Ohm Resistor
- Breadboard
- Jumper wires
You can use the preceding links to find all the parts for your projects at the best price!
Schematic Diagram
Assemble all of the parts by following the schematic diagram in the next section:
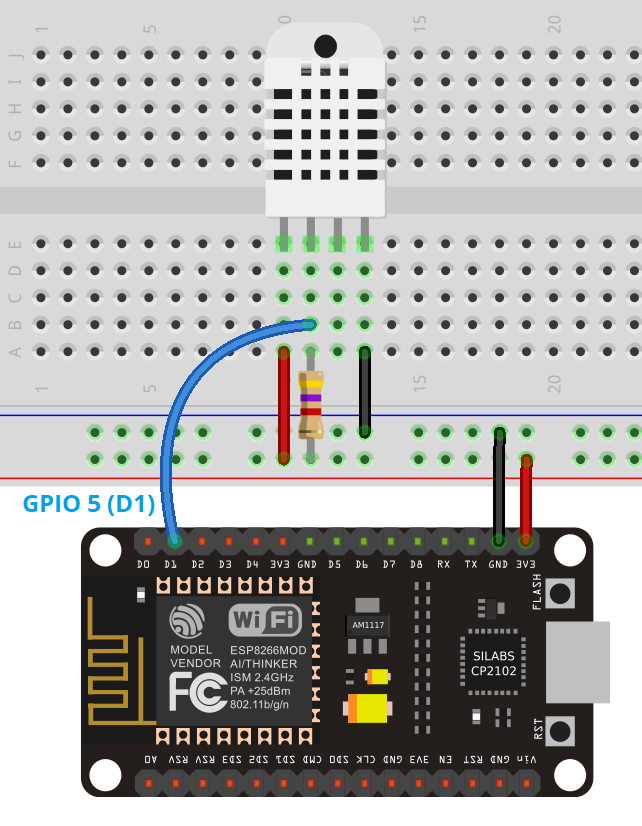
Connecting to the ESP8266 Access Point
Open your Wi-Fi settings and tap the ESP8266-Access-Point network while the ESP8266 is running the sketch on your smartphone.

Enter the password you defined earlier.
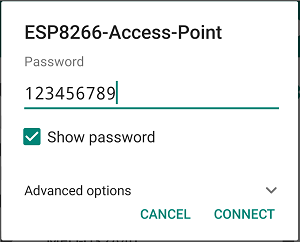
Open your web browser and type http://192.168.4.1 into the address bar. The following web server page should be loaded:
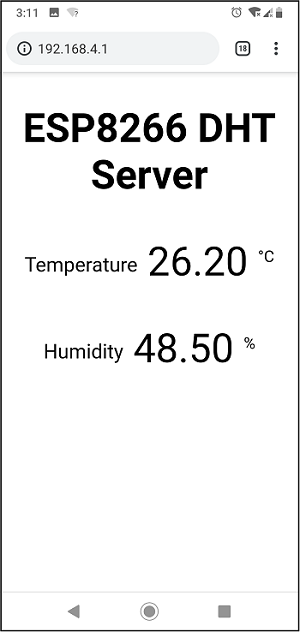
The web server page looks somewhat different from the original web server. Next to the temperature and humidity, that web server displays two web icons. The Font Awesome website is used to load those icons. However, since the ESP8266 is just a soft access point (it is not connected to the internet), we are unable to load those icons.
Conclusion
In this tutorial, you learned how to set up the ESP8266 as a soft access point. This enables you to connect directly to the ESP8266 web server through Wi-Fi without the need for a router.
Note that since the ESP8266 is not linked to the internet, you cannot perform HTTP requests to other services to publish sensor data or to get data from the internet (for example, loading the icons).
If you like ESP8266, you may also like:
- Build a Web Server with an ESP8266 to Control an RGB LED
- Build a Web Server with an ESP8266 to Control an RGB LED
- Get MAC Address of ESP32/ESP8266 and Change It (Arduino IDE)
- How to Wire an OLED Display with ESP8266 NodeMCU
We hope you find this tutorial useful. Thanks for reading.