Using the MicroPython firmware, this tutorial shows how to operate the ESP32/ESP8266 digital inputs and outputs. You'll learn, for example, how to read the value of a pushbutton and light up an LED appropriately.
Prerequisites
You need to have MicroPython firmware set up on your ESP32 or ESP8266 boards to follow this tutorial. To write the code and upload it to your board, you also need an IDE. We suggest using the uPyCraft IDE or Thonny IDE:
- Thonny IDE:
- Installing and getting started with Thonny IDE (SOON)
- Flashing MicroPython Firmware with esptool.py (SOON)
- uPyCraft IDE:
- Install uPyCraft IDE (Windows, Mac OS X, Linux)
- Flash/Upload MicroPython Firmware to ESP32 and ESP8266
We recommend following this guide if this is your first time dealing with MicroPython: Getting Started with MicroPython on ESP32 and ESP8266
Project Overview
We'll create a simple project example using a pushbutton and an LED to show you how to use digital inputs and outputs. As seen in the following image, we will read the state of the pushbutton and light it appropriately.
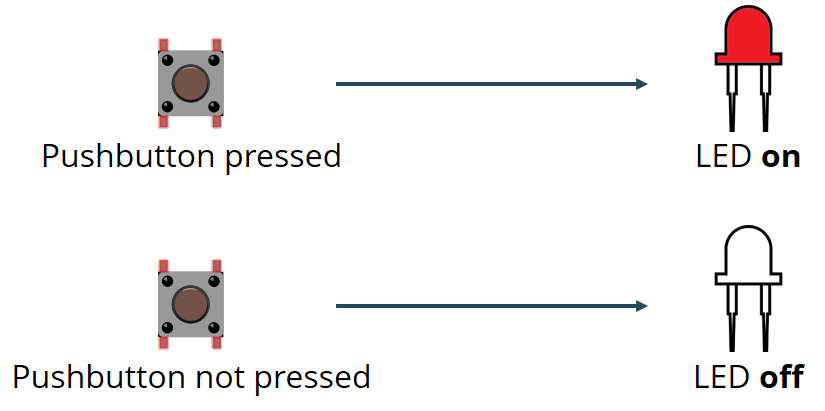
Digital Inputs
You need to first create an Pin
object and set it as an input to get the value of a GPIO. For example:
button = Pin(4, Pin.IN)
Then, you need to apply the value()
method to the Pin
object without passing any parameters to acquire its value. To access the state of an Pin
object named button
, for example, use the following expression:
button.value()
We’ll show you in more detail how everything works in the project example.
Digital Outputs
To set a GPIO on or off, first, you need to set it as an output. For example:
led = Pin(5, Pin.OUT)
Use the value()
method on the Pin
object and pass 1
or 0
as parameters to control the GPIO. The following command, for example, sets a Pin
object (led
) to HIGH:
led.value(1)
To set the GPIO to LOW, pass 0
as an argument:
led.value(0)
Schematic
You need to assemble a circuit an LED and a pushbutton before going any further. The pushbutton will be connected to GPIO 4, and the LED to GPIO 5.
Parts List
This is the hardware you'll need to complete this project:
You can use the preceding links to find all the parts for your projects at the best price!
Schematic: ESP32
If you're using an ESP32 board, follow the next schematic diagram:
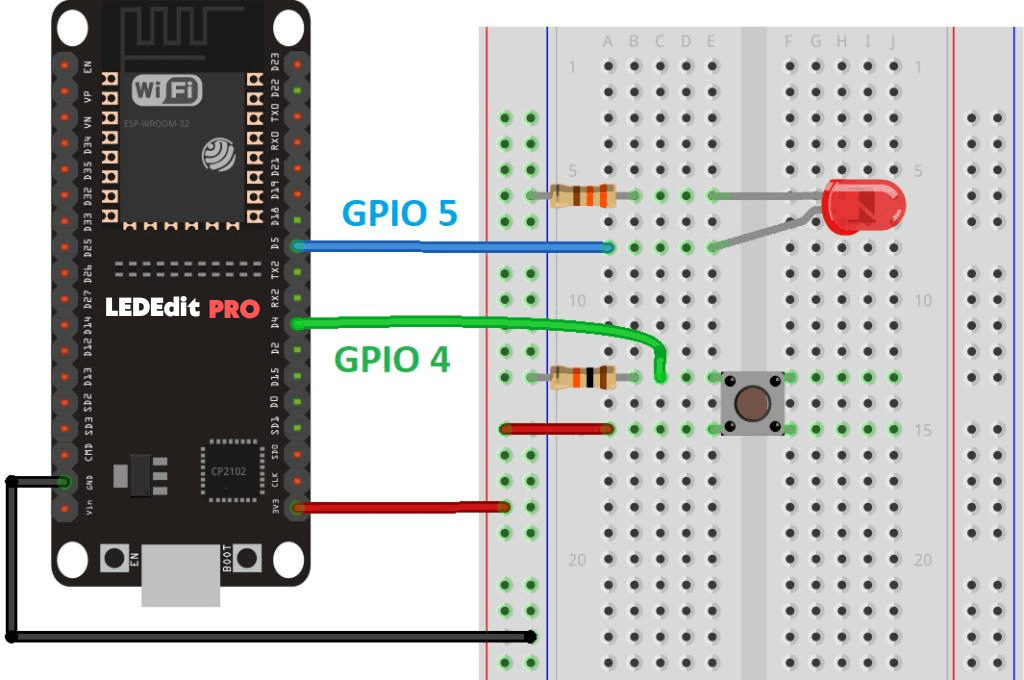
Schematic: ESP8266
If you're using an ESP8266 board, follow the next schematic diagram:
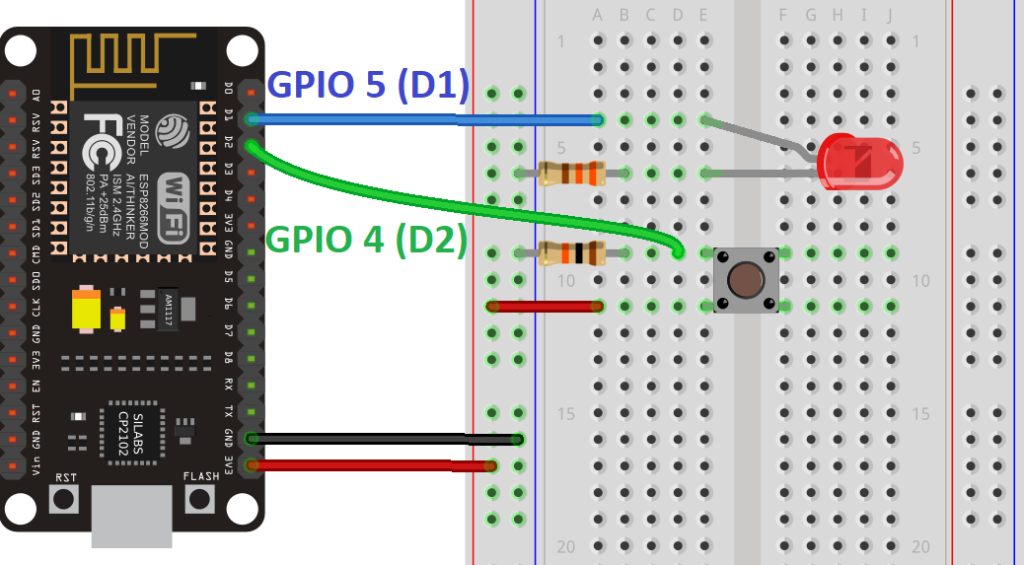
The pin marked D1 on the ESP8266 corresponds to GPIO 5, while the pin marked D2 corresponds to GPIO 4.
Script
The following code is used to read the state of the pushbutton and light up the LED appropriately. Both the ESP32 and ESP8266 boards may use the code, and it works both ways.
# Complete project details at https://lededitpro.com
from machine import Pin
from time import sleep
led = Pin(5, Pin.OUT)
button = Pin(4, Pin.IN)
while True:
led.value(button.value())
sleep(0.1)
How the Code Works
You start by importing the Pin
class from the machine
module, and the sleep
class from the time module.
from machine import Pin
from time import sleep
Then, create a Pin
object called led
on GPIO 5. LEDs are outputs, so pass as the second argument Pin.OUT
.
led = Pin(5, Pin.OUT)
We also create an object called button
on GPIO 4. Buttons are inputs, so use Pin.IN
.
button = Pin(4, Pin.IN)
Use button.value()
to return/read the button state. Then, pass the button.value()
expression as an argument to the LED value.
led.value(button.value())
This way, when we press the button, button.value()
returns 1
. So, this is the same as having led.value(1)
. This sets the LED state to 1
, lighting up the LED. When the pushbutton is not being pressed, button.value()
returns 0
. So, we have led.value(0)
, and the LED stays off.
Demonstration
Using uPyCraft IDE or Thonny IDE, save the code to your ESP board. When you press the button, the LED should then light up, and when you release it, it should stay off.
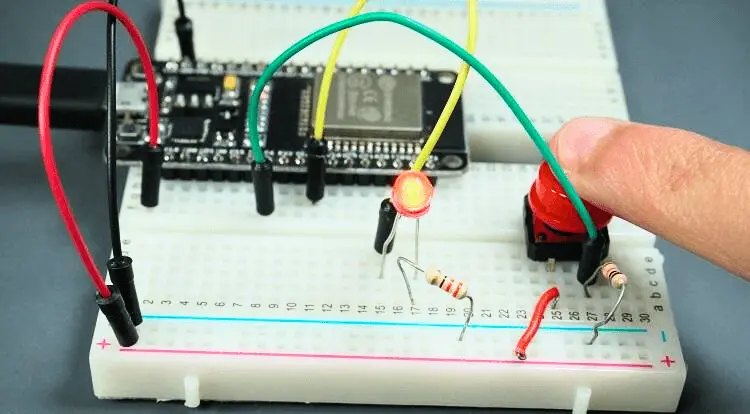
Conclusion
To wrap up, to read the value of a GPIO, we simply need to use the value()
method on the corresponding Pin
object. To set the value of a GPIO, we just need to pass as arguments 1
or 0
to the value()
method to turn it on or off, respectively.
If you like MicroPython, you may also like:
- How to Flash MicroPython Firmware to ESP32 and ESP8266
- Getting Started with MicroPython on ESP32 and ESP8266
- How to Install uPyCraft IDE on a Windows PC
We hope you find this tutorial useful. Thanks for reading.